Greeting JavaScript: If, else, else if, switch statements and ternary operator in JavaScript7 min read
On a daily basis, we often do task based on the real condition. For example, if the weather is nice today, then we can go outside and do some cool stuff. If you’re feeling hungry, then you eat some snacks. If you feel tired, then you go to sleep and so on…JavaScript and other programming languages have been created for real-life human’s demands. It’s the reason why JavaScript also has some statements that perform the conditional actions and which if
else
else if
switch
and also the ternary operator
.
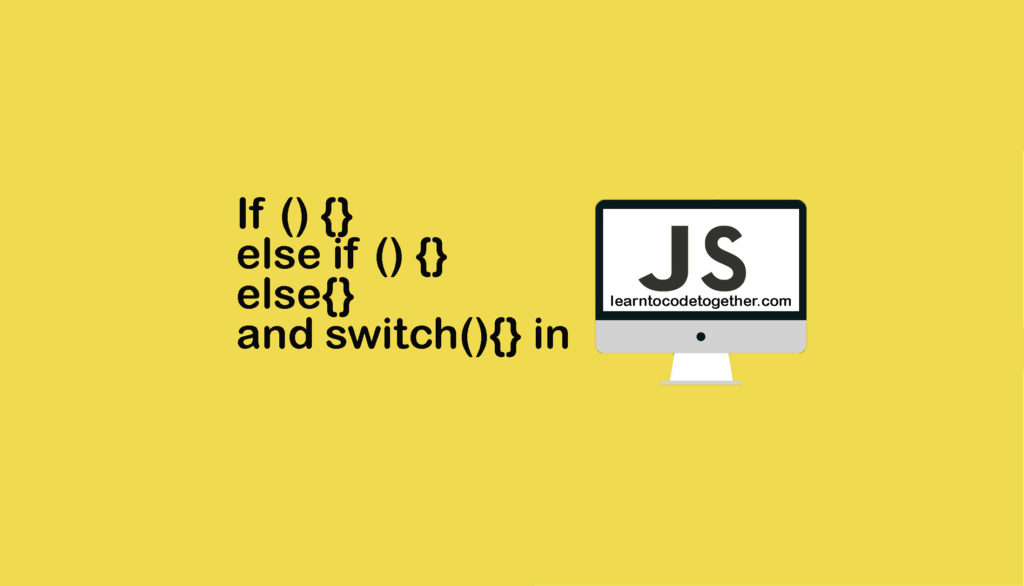
If statements
In order to get a better visualization, let’s take a look about what it looks like and its syntax first:
if (condition is true) {
statement is executed
}
It looks amiable and brief, right? So the if
‘s statements are used to make a decision in our code. The code in the curly bracket will be executed under certain conditions based on what’ve been defined in the parentheses beside if
true
true
false
false
the if
condition will never run (as you probably guess.)
Now, let’s make some particularly examples:
var x = 20;
if (x > 10){
console.log("This number is greater than 10!");
} // This number is greater than 10!
Look at the example above. First, we defined a variable named x and assign it to 20, afterward if
This number is greater than 10!
Else Statements
if
statements will be executed only when a certain condition true
if
else
statement. If something cannot happen, so else
if (condition) {
// if true, execute something
}
else {
// in case if is false, execute something
}
In case, a condition if
if
else
statement instead:
var x = 10;
if (x < 10){
console.log("X is less than 10");
}else{
console.log("X is not less than 10");
}
// X is not less than 10
With else
if
if
else
else
else
var x = 10;
if(x > 10){
console.log(x + " is greater than 10!");
}else{
if(x < 10){
console.log(x + " is less than 10!");
}else{
console.log(x + " is equal to 10!");
}
}
// Output: 10 is equal to 10!
Look at the example above, you can create a nested
Else if Statement
Sometimes you have multiple conditions that need to be addressed, but it is possible to use if else
else
if
statement together with else if
statement:
var num = 4;
if (num > 15) {
console.log("Bigger than 15");
} else if (num < 5) {
console.log("Smaller than 5");
} else {
console.log("Between 5 and 15");
}
// Output: Smaller than 5
You can add one or more or many as you else if
else
if
else if
if
The function is executed from top to the bottom, so be careful to choose what kind of condition you want to come first. For example:
var x = 5;
if (x < 7) {
console.log("Less than seven");
} else if (x < 6) {
console.log("Less than six");
} else {
console.log("Less than five");
}
}
// Output: Less than seven
Switch Statement
If sometimes you have many options and want to execute something based on keyboard command. Not if-else
switch
switch
statement tests a value and can have many case
statements which define various possible values. Statements are executed from the first matched case
value until a break
is encountered.
Let’s see switch
‘s syntax and how it looks like first:
switch (expression){
case constant1:
statement;
break;
case constant2:
statement;
break;
case constant3:
statement;
break;
default
statement;
}
Primarily, the parentheses switch
break
case
values are tested with strict equality (===
) (don’t need to worry ===
break
default
Now let’s see switch
statement in practice:
var dayInWeek = 8;
switch (dayInWeek){
case 2:
console.log("Today is Monday!");
break;
case 3:
console.log("Today is Tuesday!");
break;
case 4:
console.log("Today is Wednesday!");
break;
case 5:
console.log("Today is Thursday!");
break;
case 6:
console.log("Today is Friday!");
break;
case 7:
console.log("Today is Saturday!");
break;
default:
console.log("Today is Sunday! Whoo-weee yo");
}
// Output: Today is Sunday! Whoo-wee yo
We have a variable dayInWeek
switch
if else
switch
break
colon
case
Ternary Operator
Ternary is frequently used as a short syntax of if statement. Because of it is short, so it’s confusing sometimes. The syntax would look like this:
condition ? ifTrueDoSomething : otherwiseDoOtherThing
Let’s break thing down and see what happens:
condition
: The expression and its value is used as a conditionifTrueDoSomething
: if the expression in the condition is evaluated to a truthy value, then execute somethingotherwiseDoOtherThing
: if the condition is labeled to the falsy value, then execute something.
For example, we want to check whether the value is greater than or equals 18, then execute something, other do something else:
var age = 21;
var adultAge = 18;
console.log(age >= adultAge ? "You're an adult" : "You're not an adult");
// expected output: You're an adult
Because the condition
is labelled to a truthy value, so You're an adult
was printed out to the console.
We can rewrite this piece of code with if else
statement:
var age = 21;
var adultAge = 18;
if(age >= adultAge){
console.log("You're an adult");
}else{
console.log("You're not an adult");
}
See, ternary operator is much shorter than if else statement.
Besides false
, possible falsy expressions are: null
, NaN
, 0
, the empty string (""
), and undefined
. If condition
is any of these, the result of the conditional expression will be exprIfFalse
. (try it now on your browser)
A simple example:
var age = 26;
var beverage = (age >= 21) ? "Beer" : "Juice";
console.log(beverage); // "Beer"
Also we can chain ternary operators together, it’s much shorter than if else statement, but it leads more confusing:
function example(…) {
return condition1 ? value1
: condition2 ? value2
: condition3 ? value3
: value4;
}
// Equivalent to:
function example(…) {
if (condition1) { return value1; }
else if (condition2) { return value2; }
else if (condition3) { return value3; }
else { return value4; }
}
Conclusion
Today we’ve learned about some fundamental things in JavaScript, if, else
statement, else if
statement switch
if else
switch
boolean
value is either labeled to true
or false
, not something midground. Truthy
value is what is evaluated to true, and a falsy value is what is evaluated to false
otherwise. We’ve learned what is ternary operator, which is much shorter than traditional if else
statement.