Learn JavaScript Arrays from Scratch12 min read
In a few previous parts, we’ve been working and handling some core topics in JavaScript. We are already known that a variable is a container for value and store that value such as username, phone number, etc…in memory piece by piece. However let’s imagine now we have a shopping list and we have to print out all of them into the
Or if you came up with an idea store all of them in a variable with a string and separate them by a comma. It is not really wise because remember string is the primitive value which means immutable, you cannot alter them when you already declared (but don’t confuse with the variable assignment). There are also 5 other primitive values, which are number, boolean, null, undefined and symbol. All of 6 kinds of values are not an object, have no method and immutable (I want to mention those values just in case I have not talked about it).
So, storing the shopping list in a variable is not judicious. Do we have another way to do it more effectively, clearly? Of course, JavaScript has, that is the time when Array comes in handy, which is used to organize, maintain and store a number of elements on only one variable such as a shopping list. Suppose we have a shopping list like this:
- Apple
- Bread
- Milk
- Water
- Toothpaste
Table of Contents
Before we start
Arrays you can see it as a kind of data structure that frequently used in many applications and it is used for storing multiple values at a time. If you have learned some other programming languages before you learn JavaScript such as Java or C, you already know that in these languages, the size of an array is usually fixed, it is usually stored in a contiguous block of memory and when you declare the type of the array, then its elements have to obey this rule, if there is an array type of integer, then an element with type of double is not allowed. But it’s different in JavaScript, JavaScript’s arrays are dynamic since an array’s length can change at any time, data can be stored at non-contiguous locations in the array and you can store multiple types of data inside it. Those characteristics make it much easier to work with arrays in JavaScript.
Also read:
Create an array
Array literal
Now we have a shopping list and all the items we want to buy from the grocery store, then we can simply use an array in JavaScript to store our items like this:
var shoppingList = ["Apple", "Bread", "Milk", "Water", "Toothpaste"]; console.log(shoppingList); // ["Apple", "Bread", "Milk", "Water", "Toothpaste"]
Create an array in JavaScript is pretty straightforward, one way you can create this by an array literal. Which creates an array by wrapping items into square brackets [ ] (like the example above). Let’s break down what happened with the code example above:
- The array is created by wrapping items inside square brackets [ ].
- The array first index is started from 0, which means the first element has 0 as its index, 1 is the index of the second element and so forth.
- In the example array above, each item is an element of an array.
- Not just storing
string ,array can store different data types such as number, boolean, object, function, nested array, etc…even all of them together!
Array constructor
This is the alternative way lets you create an array in JavaScript, with kind of syntax below:
var score = new Array();
console.log(score); // []
Create a new variable and assigns to it the syntax new Array() and a new array has been initialized.
We’ve created an array with empty elements because we didn’t pass into this array any value. If you wanted to give it some values, no need to use square brackets, just pass the values you want to convey into the parentheses:
var score = new Array(1, 2, 3);
console.log(score); // [1, 2, 3];
var string = new Array("A string", true, 12, undefined, null);
console.log(string); // ["A string", true, 12, undefined, null]
But JavaScript also lets you omit the new keyword when you create an array constructor if you wanted.
var tvShow = Array("Rick and Morty", "Mr Robot", "Silicon Valley");
console.log(tvShow); // ["Rick and Morty", "Mr Robot", "Silicon Valley"]
Manipulate the Array’s Element
Array’s Length Property
In order to know how many elements are existing in your array, we use
var characters = ["Jessica", "Morty", "Noob-Noob", "Zeep", "Rick", "Scary Terry", "Beth", "Jerry", "Summer", "Poopybutthole", "BirdPerson"];
console.log(characters.length); // 11
Access Elements in an Array
Now let’s open the console and see the output of the example array:
var shoppingList = ["Apple", "Bread", "Milk", "Water", "Toothpaste"];
console.log(shoppingList);
// ["Apple", "Bread", "Milk", "Water", "Toothpaste"]
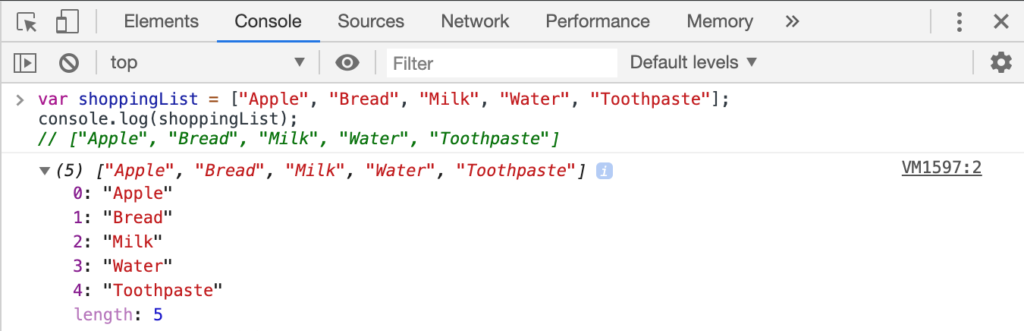
As you can see, our array has 5 items correspond with 5 elements in
shoppingList[0]; // "Apple"
// Or save the value you want to return in a new variable:
var oneElement = shoppingList[2];
console.log(oneElement); // "Milk"
Replace/Update Elements in an Array
This is the way you can access and get the value of an element in an array by using square brackets that you want, which called bracket notation. You can update the value of elements inside an array
var rainbow = ["Red", "Orange", "Black", "Green", "Blue","Indigo", "Violet"];
rainbow[2] = "Yellow";
console.log(rainbow);
// ["Red", "Orange", "Yellow", "Green", "Blue", "Indigo", "Violet"]
The third element has been replaced, “Black” to “Yellow”.
Add elements to an array
Add element to the beginning
When you want to manipulate elements on an array, such as changing elements, replace or update a new array list. You need to use
To add the element to the beginning of an array, simple we just use unshift() method, inside parentheses is/are element(s) that you want to add to the array:
var fruits = ["Orange", "Banana", "Apple"];
fruits.unshift("Grape");
console.log(fruits);
// ["Grape", "Orange", "Banana", "Apple"];
See, Grape has been added to the first position of this array. But when you use unshift(), your original array would be mutated and changed. Which we called destructive method. There are also a lot of methods that help us manipulate array but will not mutate or change the original array, which is non-destructive (I will cover some of those in this article, scroll down to see, but some others I will put away those in some upcoming more advanced articles).
Add element the end
Likewise, we have push() method to add the element to the end of the array and it will mutate your original array.
var fruits = ["Orange", "Banana", "Apple"];
fruits.push("Strawberry");
console.log(fruits);
// ["Orange", "Banana", "Apple", "Strawberry"];
Delete an element from the beginning
We utilize
var fruits = ["Orange", "Banana", "Apple"];
fruits.shift();
console.log(fruits);
// ["Banana", "Apple"];
Delete Array’s Element
Delete an element from the end
We use pop() method to remove the last element in an array and returns this element, it also changes the length of the array, alike shift(), no needs the take any value:
var fruits = ["Orange", "Banana", "Apple"];
fruits.pop();
console.log(fruits);
// ["Orange", "Banana"];
Delete, update, replace a particular element
Upwards, we added, deleted from the beginning and the end of the array, but we not yet talked about the arbitrary elements that we want to modify. We have some ways to do it, but most handy is splice() method. The splice() method changes the contents of an array by removing or replacing existing elements and/or adding new elements. However, this method is a little more complex than the previous ones, splice() method has the syntax like this:
array.splice(start, deleteCount, item1, item2...)
- start: origin with 0, pick up the position you want to splice, like array index, if you want to splice at the second element, you could do like this:
array.splice(1)
- deleteCount (optional): Define how many elements you want to delete, from the given index, eliminate left to right, for instance, delete 1 element from the index 3:
array.splice(3, 1)
- item (optional): replace or add elements to array begin with start index.
For example, we have an array:
var numbers = [2, 3, 4, 5, 6];
Our task deletes the number 4 and replaces nothing, with splice() method it can be done like this:
var numbers = [2, 3, 4, 5, 6];
var removeNumber = numbers.splice(2,1);
console.log(numbers);
// [2, 3, 5, 6]
console.log(removeNumber);
// [4]
Here we have another array:
var names = ["Eric", "Lardon", "Belinda", "Tracie"];
We want to remove the second element and replace it with “Alison” and that’s it:
var names = ["Eric", "Lardon", "Belinda", "Tracie"];
names.splice(2, 1, "Jamie");
console.log(names);
// ["Eric", "Lardon", "Jamie", "Tracie"]
With those arrays, we can easily see the index of
var characters = ["Jessica", "Morty", "Noob-Noob", "Zeep", "Rick", "Scary Terry", "Beth", "Jerry", "Summer", "Poopybutthole", "BirdPerson"];
console.log(characters.indexOf("Scary Terry")); // 5
Now let’s try to remove an element which has the value “Simon” and replace it with “Anna”:
var names = ["Jessica", "Morty", "Noob-Noob", "Zeep", "Rick", "Scary Terry", "Beth", "Jerry", "Summer", "Poopybutthole", "BirdPerson", "Simon", "Elliot", "Quincy", "Dale"];
First, we have to create a variable that checks the position of “Simon” in this array:
var simonPosition = names.indexOf("Simon");
console.log(simonPosition); // 11;
Now we have the position, we can use splice the remove it, but before we do it, we need to use if condition to make sure that the value “Simon” is presented, then splice the array to remove this element and replace it with “Anna”:
if(simonPosition > -1){
names.splice(simonPosition, 1, "Anna");
}
Now wrap all of ’em together:
var names = ["Jessica", "Morty", "Noob-Noob", "Zeep", "Rick", "Scary Terry", "Beth", "Jerry", "Summer", "Poopybutthole", "BirdPerson", "Simon", "Elliot", "Quincy", "Dale"];
var simonPosition = names.indexOf("Simon");
console.log(simonPosition);
if(simonPosition > -1){
names.splice(simonPosition, 1, "Anna");
}
console.log(names);
// ["Jessica", "Morty", "Noob-Noob", "Zeep", "Rick", "Scary Terry", "Beth", "Jerry", "Summer", "Poopybutthole", "BirdPerson", "Anna", "Elliot", "Quincy", "Dale"]
So, we did it! Sometimes just have to take a look a little bit to see the index of an element. But in most cases, it’s better for us to use indexOf() method to find out the accurate position of an element. Combining with splice() method, we removed the element with the value “Simon” and replaced it with “Anna” easily and accurately.
Link two or more different array
As I said earlier, there are two ways to manipulate with arrays. One, destructive, which means the original array will be mutable and changeable. But with the elegant of functional programming, destructive is not accepted. That is the factor why we need to use the non-destructive method instead, which is provided in JavaScript.
Concat method()
With the limitation of this article, we just mention a little bit about the non-destructive method (we will cover in deep about functional programming and non-destructive method in the later sections), which is
var array1 = [1, 2, 3];
var array2 = [4, 5, 6];
var array3 = array1.concat(array2);
console.log(array3); // [1, 2, 3, 4, 5, 6]
console.log(array1); // [1, 2, 3] // Still immutable
console.log(array2); // [4, 5, 6] // Still immutable
Array nested Array
Array, like many other objects, it can be nested. That means with one primary array, you can create the different other arrays inside it
var array = [
[1, 2, 3],
[true, false, undefined],
["a", "b", "c"]
];
This code above is the typical example of a nested array, and do you know how we call this kinda array? Nested array, or more specifically, two-dimensional array. We have the outer array which obtains 3 different arrays inside it. But the question is, how we can get the data from those arrays? For instance, we want to access the value “2” on this array below, just manipulate like many other arrays:
var array = [
[1, 2, 3],
[true, false, undefined],
["a", "b", "c"]
];
console.log(array[0][1]); //2
From outside to inside, first you need to access the first inner array which is [1, 2, 3]
by using array[0]
then access the second element of this array by array[0][1]
.
However, you still can nest many arrays as you want into an array if you wanted, but the downside is with too many arrays nested in other, it’s not easy to practice and bulky to maintain.
Conclusion
From now on, you’ve equipped for yourself the fundamental of the array in JavaScript, like how to create an array, access to its value, manipulate them, what is a nested array and what is the destructive method and non-destructive method. We will dive deeper into arrays and functional programming in some later articles, I hope to see you there. Happy coding! 🙂