Learn Java from Scratch: Introduction to Java Programming5 min read
Java is a high-level and modern programming language that was designed by Sun Microsystems in 1995 and currently owned by Oracle. Java is a general-purpose, class-based, object-oriented language and designed to have as few implementation dependencies as possible.
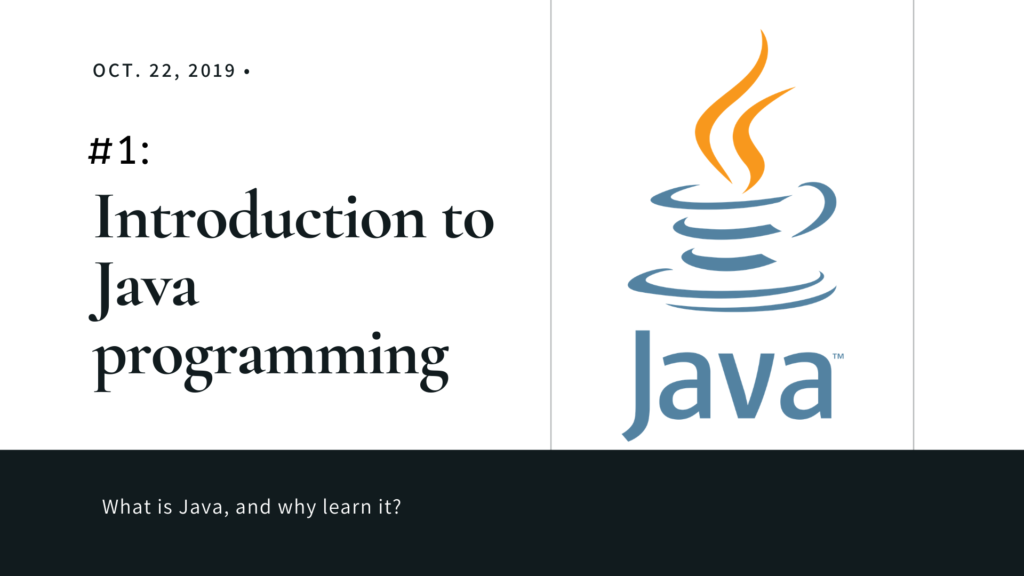
Why should I learn Java?
Java is a robust and versatile language, it can be used for many different purposes. Here are some of the main factors which make Java fashionable:
- Object-Oriented: In Java programming, you can see objects, classes everywhere. The four main concepts of object-oriented programming are Inheritance, Abstraction, Encapsulation, and Polymorphism. Don’t worry if you haven’t seen any object, class or what actually those terms are yet, because we just get started.
- Platform independent and portable: The cool thing when writing Java code is you just need to write a program once and it will be able to run on many different platforms. In other words, with Platform independent, Java guarantees that you will able to Write Once, Run Anywhere. This terminology was given by Sun Microsystems, which means the same source code could be run on any machine, Java allows run Java bytecode on any machine irrespective of the machine or the hardware thanks to JVM (Java Virtual Machine).
- Robust: Eliminating error-prone situations by emphasizing mainly on compile-time error checking and runtime checking. Garbage collection, Exception Handling, and Memory allocation are the main features that make Java is a robust language.
- Quite…simple: Learning Java at first when you haven’t come through any other OOP languages is quite struggling. Yet, when you grasp the basic concept of OOP in Java, then everything will unfold with much easier.
- Secure: In Java, you cannot access out-of-bound arrays, and you don’t have pointers, and thus several security flaws like stack corruption or buffer overflow are impossible to exploit in Java. Java also provides several tools for security, such as No buffer-overflow exploits, Byte-code verification, Security permissions for different codebases, Security-related APIs, etc…
- Multithreading: Java supports multithreading, which means two or more parts of a program can be executed concurrently at the same time for maximum utilization of CPU especially when your computer has multiple CPUs (cores).
- Distributed: Java is distributed designed for the distributed environment of the Internet.
The very first program in Java: Hello, World!
Now let’s dive in write some actual Java code, we will first make a simple Hello World program:
public class MyFirstJavaProgram {
public static void main(String[] args){
System.out.println("Hello, World!");
}
}
If you have learned some script languages such as Python or Ruby, indeed this Java code is much verbose! In the essence, classes play a critical role in any Java program, in fact, every line of code that can actually run needs to be inside a class, as you can see here we just created a class named MyFirstJavaProgram
and also you can see the keyword public
precedes the class name, this public
the keyword is a modifier working like a scope which tells Java program where it can be seen. Because it marked as public
so it’s visible to the whole Java program, it’s also a convention in Java if you define a class as public
, this class’s name and your file’s name should be the same, so here my class’s name is MyFirstJavaProgram
and hence my file’s name for this program also should be MyFirstJavaProgram.java
, otherwise, you will see some errors.
Inside this class, we have our main
method (a method is a collection of statements that are grouped together to perform an operation) which acts as an entry point to start our program, you always need to include a main method in a class in order to make this class executable. Java only starts running a program with the specific public static void main()
signature. public
, as I said before, which means it can be accessed everywhere, static
means that the method can be run without creating an instance of the class containing the main method, the void
keyword means this method will not return any value and finally main
is the name of our method.
String[] args
is a collection of String
s you pass in for processing command-line arguments. Inside our main method, we write System.out.println
statement and pass into it a string Hello, World!
which will be printed out on the screen, and remember in Java, each line of code must be ended with a semicolon. System.out.print
means after your text displayed, the mouse cursor will right next to your text, with System.out.println
, the cursor will move to the new line after your text displayed on the screen.
Conclusion
Java is kind of a must-learn programming language if you want to become a great software developer, in this article, we’ve comprehended many advantages in Java programming, and the important part of Java language is it’s an object-oriented language – and this is what makes Java popular. Write Once Run Anywhere is a cool feature of Java language, we just need to write a Java program once and expect runs everywhere. Your Java code should be written in a class or classes and need a main method to run this program. Everything may seem a little overwhelming at first, but hang in there, the more you learn, the more things will be clarified.